springboot 使用 Spring Data Elasticsearch 创建索引、判断索引存在、删除索引 作者:马育民 • 2021-09-19 23:31 • 阅读:11177 上接:[springboot 使用 Spring Data Elasticsearch 操作 Elasticsearch 7.9-创建工程](https://www.malaoshi.top/show_1IX1tKpTSEXs.html "springboot 使用 Spring Data Elasticsearch 操作 Elasticsearch 7.9-创建工程") # 说明 对索引进行操作:创建索引、判断索引是否存在、删除索引 ### controller ``` package day1.ctrl; import day1.entity.Toutiao; import org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate; import org.springframework.data.elasticsearch.core.IndexOperations; import org.springframework.data.elasticsearch.core.document.Document; import org.springframework.data.elasticsearch.core.mapping.IndexCoordinates; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import javax.annotation.Resource; import java.util.HashMap; import java.util.LinkedHashMap; import java.util.Map; @RestController() @RequestMapping("/testIndex") public class TestIndexCtrl { //注入 ElasticsearchRestTemplate @Resource private ElasticsearchRestTemplate elasticsearchRestTemplate; /** * 根据实体类创建索引 */ @RequestMapping("/create") public boolean create(){ elasticsearchRestTemplate.indexOps(Toutiao.class); return true; } /** * 根据 json 创建索引,注意不包含 mapping */ @RequestMapping("/create2") public boolean create2(){ String json="{\"properties\":{\"author\":{\"type\":\"keyword\"},\"content\":{\"type\":\"text\"},\"date\":{\"type\":\"date\"},\"like\":{\"type\":\"integer\"},\"type\":{\"type\":\"keyword\"},\"title\":{\"type\":\"text\",\"fields\":{\"keyword\":{\"type\":\"keyword\",\"ignore_above\":256}}}}}"; Document doc=Document.parse(json); IndexOperations io=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v6")); boolean b1 = io.create(); boolean b2 = io.putMapping(doc); return b1 && b2; } @RequestMapping("/create3") public boolean create3(){ /* 通过map构建出映射json结构 可指定索引名 */ Map mapping=new HashMap(); Map properties=new LinkedHashMap(); mapping.put("properties",properties); Map author=new LinkedHashMap(); author.put("type","keyword"); properties.put("author",author); Map content=new LinkedHashMap(); content.put("type","text"); content.put("analyzer","ik_smart"); properties.put("content",content); Map date=new LinkedHashMap(); date.put("type","date"); properties.put("date",date); Map like=new LinkedHashMap(); like.put("type","integer"); properties.put("like",like); Map title=new LinkedHashMap(); title.put("type","text"); title.put("analyzer","ik_smart"); properties.put("title",title); Map titleFields=new LinkedHashMap(); Map titleFieldsKeyword=new LinkedHashMap(); titleFieldsKeyword.put("type","keyword"); titleFieldsKeyword.put("ignore_above",256); titleFields.put("keyword",titleFieldsKeyword); title.put("fields",titleFields); Document doc=Document.from(mapping); IndexOperations io=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v4")); boolean b1 = io.create(); boolean b2 = io.putMapping(doc); return b1 && b2; } /** * 查看某索引映射 */ @RequestMapping("/showMapping") public Map showMapping(String index){ Map map=elasticsearchRestTemplate.indexOps(IndexCoordinates.of(index)).getMapping(); return map; } @RequestMapping("/exists") public boolean exists(String index){ //不要使用 elasticsearchRestTemplate.indexOps(Toutiao.class),会自动创建 boolean b=elasticsearchRestTemplate.indexOps(IndexCoordinates.of(index)).exists(); return b; } /** * 删除索引 * @param index */ @RequestMapping("/delete") public boolean delete(String index){ boolean b = elasticsearchRestTemplate.indexOps(IndexCoordinates.of(index)).delete(); return b; } } ``` ### 测试类 本例使用 junit 实现,在 `test` 目录下创建类,如下图: [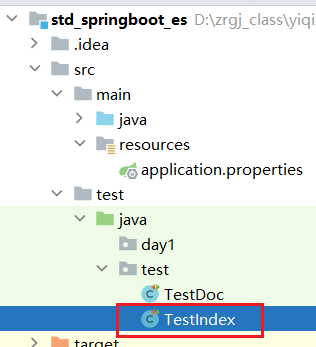](/upload/0/0/1IX4VbZvOt20.png) ``` package test; import day1.Main; import day1.entity.Toutiao; import org.junit.Test; import org.junit.runner.RunWith; import org.springframework.boot.test.context.SpringBootTest; import org.springframework.data.elasticsearch.core.ElasticsearchRestTemplate; import org.springframework.data.elasticsearch.core.IndexOperations; import org.springframework.data.elasticsearch.core.document.Document; import org.springframework.data.elasticsearch.core.mapping.IndexCoordinates; import org.springframework.test.context.junit4.SpringRunner; import javax.annotation.Resource; import java.util.HashMap; import java.util.LinkedHashMap; import java.util.Map; @RunWith(SpringRunner.class) @SpringBootTest(classes = Main.class) public class TestIndex { //注入 ElasticsearchRestTemplate @Resource private ElasticsearchRestTemplate elasticsearchRestTemplate; /** * 根据实体类创建索引 */ @org.junit.Test public void create(){ elasticsearchRestTemplate.indexOps(Toutiao.class); System.out.println("创建索引"); } /** * 根据 json 创建索引,注意不包含 mapping */ @Test public void create2(){ String json="{\"properties\":{\"author\":{\"type\":\"keyword\"},\"content\":{\"type\":\"text\"},\"date\":{\"type\":\"date\"},\"like\":{\"type\":\"integer\"},\"type\":{\"type\":\"keyword\"},\"title\":{\"type\":\"text\",\"fields\":{\"keyword\":{\"type\":\"keyword\",\"ignore_above\":256}}}}}"; Document doc=Document.parse(json); IndexOperations io=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v6")); io.create(); io.putMapping(doc); System.out.println("创建索引"); } @Test public void create3(){ //查看某索引映射 // Map map=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v6")).getMapping(); /* 通过map构建出映射json结构 可指定索引名 */ Map mapping=new HashMap(); Map properties=new LinkedHashMap(); mapping.put("properties",properties); Map author=new LinkedHashMap(); author.put("type","keyword"); properties.put("author",author); Map content=new LinkedHashMap(); content.put("type","text"); content.put("analyzer","ik_smart"); properties.put("content",content); Map date=new LinkedHashMap(); date.put("type","date"); properties.put("date",date); Map like=new LinkedHashMap(); like.put("type","integer"); properties.put("like",like); Map title=new LinkedHashMap(); title.put("type","text"); title.put("analyzer","ik_smart"); properties.put("title",title); Map titleFields=new LinkedHashMap(); Map titleFieldsKeyword=new LinkedHashMap(); titleFieldsKeyword.put("type","keyword"); titleFieldsKeyword.put("ignore_above",256); titleFields.put("keyword",titleFieldsKeyword); title.put("fields",titleFields); Document doc=Document.from(mapping); IndexOperations io=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v4")); io.create(); io.putMapping(doc); System.out.println("创建索引"); } /** * 查看某索引映射 */ @Test public void showMapping(){ Map map=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v1")).getMapping(); System.out.println(map); } @org.junit.Test public void exists(){ //不要使用 elasticsearchRestTemplate.indexOps(Toutiao.class),会自动创建 boolean b=elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v4")).exists(); System.out.println(b); } @org.junit.Test public void delete(){ //删除索引 boolean b = elasticsearchRestTemplate.indexOps(IndexCoordinates.of("toutiao_v4")).delete(); System.out.println("删除索引 = " + b); } } ``` 原文出处:http://malaoshi.top/show_1IX1tORTVEZI.html